GSAP Animation
Wednesday, January 10, 2024
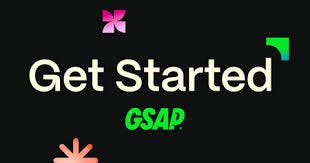
What is GSAP?
GSAP is a framework-agnostic JavaScript animation library that turns devs into animation superheroes.
Build high-performance animations that work
in every major browser. Animate CSS, SVG, canvas, React, Vue, WebGL, colors, strings, motion paths, generic objects...anything JavaScript can touch!
GSAP is unmatched in delivering advanced sequencing, reliability, and precise control for animations on over 12 million websites.
It effortlessly handles browser inconsistencies, ensuring your animations work seamlessly.
GSAP is a fast property manipulator, updating values over time with precision, and it's up to 20 times faster than jQuery!
https://jsmastery.pro JavaScript Mastery
GSAP Setup
To use GSAP, you have multiple options for integrating it into your project.
1. NPM
One common approach is to install GSAP via npm, which allows you to manage dependencies efficiently within your project's ecosystem.
This method is particularly useful for larger projects or those using modern build tools like Webpack or Parcel.
2. CDN
Alternatively, you can opt for the quick and easy
method of including GSAP directly via a CDN (Content Delivery Network) link in your HTML file.
This approach is convenient for smaller projects or when you want to quickly prototype an idea.
https://jsmastery.pro JavaScript Mastery
GSAP Setup
3. React
If you're working with React, you have the option to use the @gsap/react library, which provides seamless integration of GSAP with React components.
This allows you to harness the power of GSAP within your React applications while leveraging the component-based architecture of React.
Overall, the flexibility of GSAP's integration options ensures that you can easily incorporate it into your preferred development workflow, whether you're building a traditional website, a single-page application, or a complex web application with React.
https://jsmastery.pro JavaScript Mastery
GSAP Setup
3. React
If you're working with React, you have the option to use the @gsap/react library, which provides seamless integration of GSAP with React components.
This allows you to harness the power of GSAP within your React applications while leveraging the component-based architecture of React.
Overall, the flexibility of GSAP's integration options ensures that you can easily incorporate it into your preferred development workflow, whether you're building a traditional website, a single-page application, or a complex web application with React.
https://jsmastery.pro JavaScript Mastery
CDN Setup
To get started with GSAP, we first need to add the GSAP library to our HTML file. You can do this by grabbing the CDN link to the GSAP library.
Here’s how it will look, but with the full link.
<script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.12.... integrity="sha512-EZI2cBcGPnmR89wTgVnN3602Yyi7muWo8say... crossorigin="anonymous" referrerpolicy="no-referrer"
></script>
Make sure to place this script tag before the script tag containing your local JS file. This ensures that your local file has access to the GSAP library and its functionality.
Also, if you're using any GSAP plugins, ensure that their script tags are placed after the GSAP script tag.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
GSAP is incredibly flexible; you can use it anywhere you like, and it has zero dependencies.
If you've used any version of GSAP in the past couple of years or are familiar with tools like TweenLite, TweenMax, TimelineLite, and TimelineMax, you'll notice that in the new version, GSAP 3.0, they have all been replaced by the GSAP object.
Think of the GSAP object as the main hub for everything in GSAP.
It's like a toolbox filled with all the tools you need to create and control Tweens and Timelines, which are the main things you'll be working with in GSAP.
To really get the hang of GSAP, it's important to understand Tweens and Timelines:
https://jsmastery.pro JavaScript Mastery
GSAP Basics
Understanding Tween
Think of a Tween as the magic that makes things move smoothly—it's like a super-efficient property setter. You give it targets (the objects you want to animate), set a duration, and specify which properties you want to change.
Then, as the Tween progresses, it calculates and applies the property values at each step, creating seamless animation.
Here are some common methods for creating a Tween4
5 gsap.to(K
5 gsap.from(K
5 gsap.fromTo()
https://jsmastery.pro JavaScript Mastery
GSAP Basics
For simple animations (no fancy sequencing), the methods above are all you need! For example:
gsap.to(".box", { rotation: 27, x: 100, duration: 1 });
rotate and move elements with a class of "box"
("x" is a shortcut for a translateX() transform) over the duration of 1 second.
Basic sequencing can be achieved by utilizing the delay special property.
Let's take a closer look at the syntax.
method
target
gsap.to(".box", { rotation: 27 });
https://jsmastery.pro
JavaScript Mastery
vars
GSAP Basics
We've got a method, a target and a vars object which all contain information about the animation
The method(s)
There are four types of tweens:
gsap.to()
his is the most common type of tween. A to() tween starts at the element's current state and animates "to" the values defined in the tween.
gsap.from()
This is similar to a backwards to() tween. It animates "from" the values defined in the tween and ends at the element's current state.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
gsap.fromTo()
With this method, you define both the starting and ending values for the tween.
gsap.set()
This method immediately sets properties without any animation. It's essentially a zero-duration to() tween.
These methods provide flexibility in how you create tweens and allow you to achieve various effects in your animations.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
The target(s)
In GSAP, you need to specify what you want to animate, known as the target or targets. GSAP internally utilizes document.querySelectorAll(), allowing you to use selector text like ".class" or "#id" for HTML or SVG targets. Alternatively, you can pass in a variable or an Array.
Here are some examples:
// Using a class or ID gsap.to(".box", { x: 500 });
// Using a complex CSS selector gsap.to("section > .box", { x: 900 });
// Using a variable let box = document.querySelector(".box"); gsap.to(box, { x: 200 });
// Using an Array of elements let square = document.querySelector(".square"); let circle = document.querySelector(".circle"); gsap.to([square, circle], { x: 200 });
https://jsmastery.pro
JavaScript Mastery
GSAP Basics
These examples showcase how you can specify different targets for your animations in GSAP, allowing you to animate various elements with different properties and values.
The variables
The vars object holds all the details about the animation. It includes properties you wish to animate, as well as special properties that control the animation's behavior, such as duration, or repeat.
Here's how you might use it in a gsap.to() tween:
gsap.to(target, { // This is the vars object // It contains properties to animate x: 200, rotation: 360,
// Along with special properties
duration: 2, });
https://jsmastery.pro
JavaScript Mastery
GSAP Basics
What properties can we animate?
With GSAP, you have the flexibility to animate almost anything you can imagine. There's no predefined list of properties you can animate because GSAP is incredibly versatile.
You can animate CSS properties like width, height, color, and font-size, as well as custom object properties. GSAP even allows you to animate CSS variables and complex strings!
While you can animate virtually any property, some of the most commonly animated properties include transforms (such as translate, rotate, scale), opacity, and position. These properties are frequently used to create smooth and visually appealing animations across various elements on your webpage.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
However, for more advanced sequencing and complex choreography, Timelines are the way to go. They make the process much easier & more intuitive.
Understanding Timeline
A Timeline serves as a container for Tweens, making it the ultimate tool for sequencing animations. With a Timeline, you have the power to position animations in time exactly where you want them.
You can effortlessly control the entire sequence using methodslikepause(), play(), progress(), reverse(), and timeScale(), among others.
The beauty of Timelines is that you can create as many as you need, and even nest them, which is great for organizing your animation code into manageable modules.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
Here's the cool part: every animation (whether it's a Tween or another Timeline) is placed onto a parent timeline (which is usually the globalTimeline by default).
This means that when you move a Timeline's playhead, it cascades down through its children, ensuring that all the animations stay perfectly synchronized.
It's important to note that a Timeline is all about grouping & coordinating animations in time, it doesn't actually set properties on targets like Tweens do.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
To create a Timeline in GSAP, you simply use the method:
gsap.timeline()
With GSAP's API, you have the power to control virtually anything on-the-fly.
You can manipulate the playhead position, adjust the startTime of any child, play, pause, or reverse animations, alter the timeScale, and much more.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
Sequencing things in a Timeline
To create a Timeline, you first initialize it like this:
var tl = gsap.timeline();
Then, you can add tweens using one of the convenience methods like to(),from(),or fromTo():
tl.to(".box", { duration: 2, x: 100, opacity: 0.5 });
You can repeat this process as many times as needed. Notice that we're calling .to() on the timeline instance (in this case, the variable tl), not on the gsap object.
This creates a tween and immediately adds it to that specific Timeline.
https://jsmastery.pro JavaScript Mastery
GSAP Basics
By default, the animations will be sequenced one- after-the-other. You can even use method chaining to simplify your code:
// Sequenced one-after-the-other tl.to(".box1", { duration: 2, x: 100 }) .to(".box2", { duration: 1, y: 200 })
.to(".box3", { duration: 3, rotation: 360 });
It's worth noting that while you could create individual tween instances with gsap.to() and then use timeline.add() to add each one, it's much easier to call .to(), .from(),or.fromTo()directlyonthe Timeline instance.
This approach accomplishes the same thing in fewer steps, keeping your code clean and concise.
https://jsmastery.pro JavaScript Mastery
React GSAP
Why choose GSAP with React?
While React-specific libraries provide a declarative approach to animation, GSAP offers unique advantages.
Animating imperatively with GSAP empowers you with greater control, flexibility, and creativity.
Whether you're animating DOM elements, SVGs, three.js, canvas, or WebGL, GSAP allows you to unleash your imagination without limits.
What sets GSAP apart is its framework-agnostic nature. This means that your animation skills seamlessly transfer to any project, be it Vanilla JS, React, Vue, Angular, or Webflow.